Social Media Messaging Connector Integration Guide
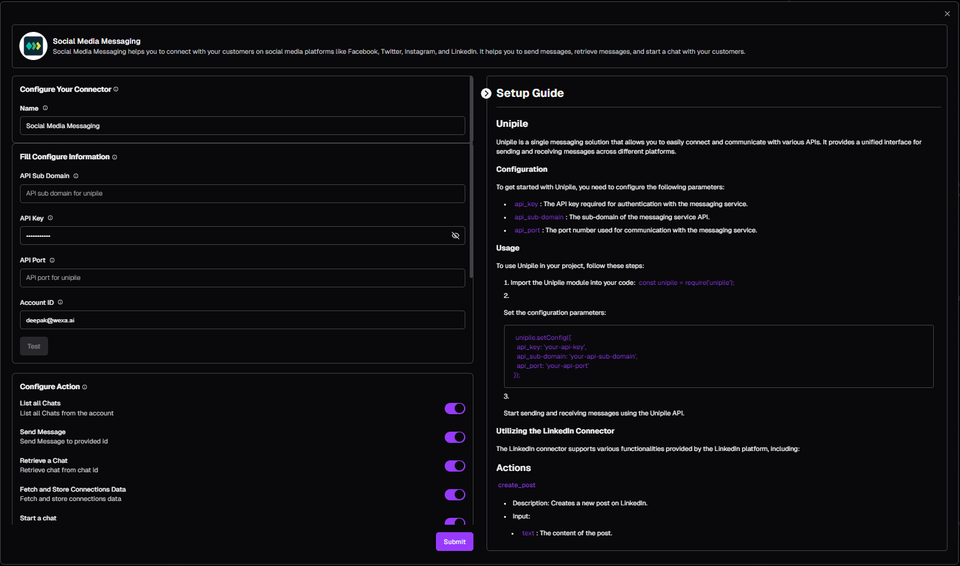
Introduction
Unipile is a single messaging solution that allows you to easily connect and communicate with various APIs. It provides a unified interface for sending and receiving messages across different platforms.
Configuration
To get started with Unipile, you need to configure the following parameters:
api_key
: The API key required for authentication with the messaging service.api_sub-domain
: The sub-domain of the messaging service API.api_port
: The port number used for communication with the messaging service.
Usage
To use Unipile in your project, follow these steps:
- Import the Unipile module into your code:
const unipile = require('unipile');
- Start sending and receiving messages using the Unipile API.
Set the configuration parameters:
unipile.setConfig({
api_key: 'your-api-key',
api_sub-domain: 'your-api-sub-domain',
api_port: 'your-api-port'
});
Utilizing the LinkedIn Connector
The LinkedIn connector supports various functionalities provided by the LinkedIn platform, including:
Actions
create_post
- Description: Creates a new post on LinkedIn.
- Input:
text
: The content of the post.
- Output:
post_id
: The ID of the created post.status
: The status of the post creation.
fetch_connections
- Description: Fetches connections from LinkedIn.
- Input:
limit
: Optional, default is "100".table_name
: The name of the table to store connections.
- Output:
message
: Status message of the operation.
list_all_chats
- Description: Lists all chats from LinkedIn.
- Input:
account_type
: The type of account (e.g., LINKEDIN, TWITTER, etc.).is_unread
: Optional, default is "false".before_timeline
: Optional.after_timeline
: Optional.limit
: Optional.
- Output:
accounts
: Array of accounts.
list_all_comments
- Description: Lists all comments on a post.
- Input:
post_id
: The ID of the post.limit
: Optional.
- Output:
comments
: Array of comments.
list_all_invitations
- Description: Lists all invitations.
- Input:
limit
: Optional.
- Output:
invitations
: Array of invitations.
list_all_messages
- Description: Lists messages in a chat.
- Input:
chat_id
: Optional.before_timeline
: Optional.after_timeline
: Optional.limit
: Optional.sender_id
: Optional.chat_index
: Optional.
- Output:
messages
: Array of messages.
list_all_posts
- Description: Lists all posts.
- Input:
provider_id
: Optional.linkedin_url
: Optional.is_company
: Optional, default is "false".limit
: Optional.cursor
: Optional, default is "1".
- Output:
posts
: Array of posts.
list_all_reactions
- Description: Fetches reactions on a post.
- Input:
post_id
: Optional.limit
: Optional.cursor
: Optional.post_index
: Optional.linkedin_url
: Optional.
- Output:
reactions
: Array of reactions.
list_attendee_messages
- Description: Lists messages from an attendee.
- Input:
attendee_id
: The ID of the attendee.cursor
: Optional.before_timeline
: Optional.after_timeline
: Optional.limit
: Optional.
- Output:
messages
: Array of messages.
list_attendees_from_chat
- Description: Lists attendees from a chat.
- Input:
limit
: Optional.chat_id
: Optional.chat_index
: Optional.
- Output:
attendees
: Array of attendees.
list_relations
- Description: Lists relations.
- Input:
limit
: Optional.
- Output:
relations
: Array of relations.
post_comment
- Description: Posts a comment on a post.
- Input:
post_id
: The ID of the post.text
: The content of the comment.
- Output:
comment_id
: The ID of the comment.status
: The status of the comment posting.
post_reaction
- Description: Posts a reaction on a post.
- Input:
post_id
: The ID of the post.reaction_type
: The type of reaction (e.g., like).
- Output:
reaction_id
: The ID of the reaction.status
: The status of the reaction posting.
profile_company_retrieve
- Description: Retrieves a company profile.
- Input:
profile_linkedin_url
: The LinkedIn URL of the profile.
- Output:
data
: The company profile data.
retrieve_all_profiles
- Description: Retrieves all profiles.
- Input:
identifiers
: List of profile identifiers.linkedin_sections
: Optional, list of LinkedIn sections to retrieve.
- Output:
profiles_data
: Array of profile data.
retrieve_attendee
- Description: Retrieves an attendee.
- Input:
chat_id
: The ID of the chat.
- Output:
attendees
: Array of attendees.
retrieve_chat
- Description: Retrieves a chat.
- Input:
chat_id
: Optional.chat_index
: Optional.
- Output:
chat_id
: The ID of the chat.chat_content
: The content of the chat.
retrieve_company_profile
- Description: Retrieves a company profile.
- Input:
company_id
: Optional.linkedin_url
: Optional.
- Output:
company_profile
: The company profile data.
retrieve_message
- Description: Retrieves a message.
- Input:
message_id
: The ID of the message.
- Output:
message_id
: The ID of the message.message_content
: The content of the message.timestamp
: The timestamp of the message.
retrieve_profile
- Description: Retrieves a profile.
- Input:
identifier
: The identifier of the profile.linkedin_sections
: Optional, list of LinkedIn sections to retrieve.
- Output:
profile
: The profile data.
send_invitation
- Description: Sends an invitation.
- Input:
provider_id
: Optional.message
: Optional.linkedin_url
: Optional.
- Output:
invitation_id
: The ID of the invitation.status
: The status of the invitation.message
: The message of the invitation.
send_message
- Description: Sends a message.
- Input:
chat_id
: Optional.chat_index
: Optional.text
: The content of the message.thread_id
: Optional.
- Output:
message_id
: The ID of the message.status
: The status of the message.text
: The content of the message.
start_chat
- Description: Starts a new chat.
- Input:
attendees_ids
: Optional.text
: The content of the chat.linkedin_url
: Optional.
- Output:
chat_id
: The ID of the chat.status
: The status of the chat.message
: The message of the chat.
withdraw_invitation
- Description: Withdraws an invitation.
- Input:
invitation_id
: The ID of the invitation.
- Output:
message
: The status message of the operation.
Contributing
Contributions are welcome! If you find any issues or have suggestions for improvements, please open an issue or submit a pull request on the Unipile GitHub repository.
License
Unipile is released under the MIT License.